Python Basic Operators: 10 Practical Examples
In this tutorial on “Python Basic Operators: 10 Practical Examples“, we will learn the basic operators with examples. Python, a versatile and powerful programming language, is favored by beginners and professionals alike for its readability and simplicity. One of the foundational aspects of Python, and indeed any programming language, is the understanding of operators. Operators are special symbols that carry out arithmetic or logical computation. In this blog post, we’ll explore 10 practical examples of basic operators in Python, covering arithmetic, comparison, assignment, logical, and identity operators. Let’s dive in!
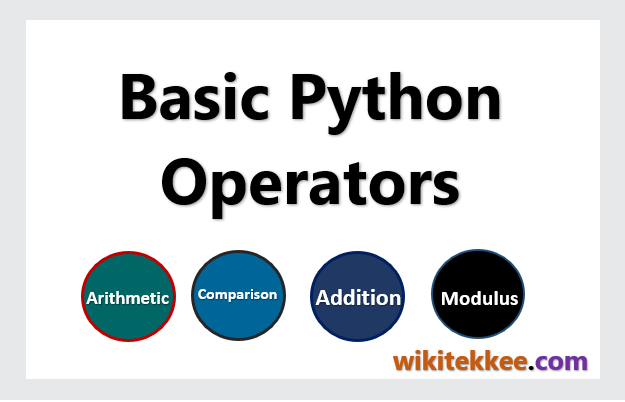
1. Arithmetic Operators:
Python, like any programming language, supports various arithmetic operators that allow you to perform mathematical calculations on numbers. These operators are fundamental to any kind of numerical manipulation in Python, from basic arithmetic to more complex mathematical operations. In this article, we’ll explore the different arithmetic operators available in Python, providing examples for each to illustrate their usage.
1. Addition (+):
Addition is one of the fundamental arithmetic operations, and in Python, it’s as straightforward as it gets. The addition operator (+
) not only allows us to perform arithmetic to add numbers but also extends its utility to concatenate strings, lists, and more. In this article, we’ll explore 5 practical examples to demonstrate the versatility of the addition operator in Python.
Examples of Addition Operator:
1.1. Adding Integers
The most basic use of the addition operator is to add two or more integers. This operation returns the sum of the numbers.
# Example 1: Adding integers
a = 5
b = 3
sum = a + b
print(f"The sum of {a} and {b} is {sum}.")
# Output: The sum of 5 and 3 is 8.
1.2. Adding Floating-Point Numbers
Addition in Python is not limited to integers; it works seamlessly with floating-point numbers (decimals) as well.
# Example 2: Adding floating-point numbers
a = 2.5
b = 3.5
sum = a + b
print(f"The sum of {a} and {b} is {sum}.")
# Output: The sum of 2.5 and 3.5 is 6.0.
1.3. Concatenating Strings
The addition operator can be used to concatenate strings, effectively joining them together into a single string.
# Example 3: Concatenating strings
first_name = "John"
last_name = "Doe"
full_name = first_name + " " + last_name
print(f"The full name is {full_name}.")
# Output: The full name is John Doe.
1.4. Merging Lists
Similar to strings, lists can also be concatenated using the addition operator, resulting in a new list that combines the elements of both lists.
# Example 4: Merging lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
merged_list = list1 + list2
print(f"The merged list is {merged_list}.")
# Output: The merged list is [1, 2, 3, 4, 5, 6].
1.5. Adding Elements of a List
While the addition operator does not directly add up the elements of a list, the built-in sum
function can be used to achieve this, showcasing the versatility of addition in Python.
# Example 5: Adding elements of a list
numbers = [1, 2, 3, 4, 5]
total_sum = sum(numbers)
print(f"The sum of the numbers list is {total_sum}.")
# Output: The sum of the numbers list is 15.
2. Subtraction (-):
Subtraction is a fundamental arithmetic operation that is as essential as addition. In Python, the subtraction operator (-
) allows us to perform arithmetic subtraction between numbers, calculate differences, and even manipulate data structures in certain contexts. This article will delve into 5 practical examples to showcase the versatility and utility of the subtraction operator in Python.
2.1. Subtracting Integers
The most straightforward use of the subtraction operator is to subtract one integer from another. This operation results in the difference between the two numbers.
# Example 1: Subtracting integers
a = 10
b = 4
difference = a - b
print(f"The difference between {a} and {b} is {difference}.")
# Output: The difference between 10 and 4 is 6.
2.2. Subtracting Floating-Point Numbers
Subtraction in Python works seamlessly with floating-point numbers (decimals) as well, allowing for precision arithmetic.
# Example 2: Subtracting floating-point numbers
a = 5.5
b = 2.2
difference = a - b
print(f"The difference between {a} and {b} is {difference}.")
# Output: The difference between 5.5 and 2.2 is 3.3.
2.3. Calculating Time Difference
Subtraction can be used to calculate the difference between times, which is particularly useful in time management and scheduling applications.
# Example 3: Calculating time difference
from datetime import datetime
time_start = datetime(2023, 4, 1, 8, 30)
time_end = datetime(2023, 4, 1, 12, 45)
time_difference = time_end - time_start
print(f"The time difference is {time_difference}.")
# Output: The time difference is 4:15:00.
2.4. Finding the Change in Value
In finance and data analysis, subtraction is used to calculate the change in value over time, such as the change in stock prices or temperature variations.
# Example 4: Finding the change in value
stock_price_yesterday = 150
stock_price_today = 155
price_change = stock_price_today - stock_price_yesterday
print(f"The stock price changed by ${price_change} since yesterday.")
# Output: The stock price changed by $5 since yesterday.
2.5. Manipulating Lists (Advanced)
While the subtraction operator is not directly used to manipulate lists, understanding how to achieve a similar effect can be useful. For example, removing elements from a list can be thought of as “subtracting” elements. This requires more advanced techniques such as list comprehensions or using the filter
function.
# Example 5: "Subtracting" elements from a list (Advanced)
original_list = [1, 2, 3, 4, 5]
elements_to_remove = [2, 4]
# Using list comprehension to "subtract" elements
resulting_list = [item for item in original_list if item not in elements_to_remove]
print(f"List after 'subtraction': {resulting_list}.")
# Output: List after 'subtraction': [1, 3, 5].
3. Multiplication (*):
The multiplication operator, denoted by an asterisk (*
), is one of the fundamental arithmetic operators in Python. It allows you to calculate the product of two numbers, perform repeated addition, and even manipulate data structures like strings and lists in interesting ways. Here are five examples that showcase the versatility of the multiplication operator in Python.
3.1. Multiplying Numeric Values
The most straightforward use of the multiplication operator is to multiply two numeric values, whether they are integers or floating-point numbers.
# Multiplying two integers
result = 7 * 5
print(result) # Output: 35
# Multiplying a float and an integer
result = 3.5 * 2
print(result) # Output: 7.0
3.2. Repeating Strings
The multiplication operator can be applied to strings. When used with a string and an integer, it repeats the string the specified number of times.
# Repeating a string
message = "Hello! " * 3
print(message) # Output: Hello! Hello! Hello!
3.3. Expanding Lists
Similarly, you can use the multiplication operator to repeat the contents of a list. This can be a quick way to initialize a list with a repeated pattern or value.
# Repeating elements in a list
numbers = [1, 2, 3] * 2
print(numbers) # Output: [1, 2, 3, 1, 2, 3]
3.4. Matrix Multiplication with NumPy
In more advanced applications, such as linear algebra, the multiplication operator can be used in conjunction with libraries like NumPy to perform matrix multiplication.
import numpy as np
# Matrix multiplication
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
result = A @ B # or np.dot(A, B)
print(result)
# Output:
# [[19 22]
# [43 50]]
3.5. Multiplying Elements in a List
While Python’s multiplication operator doesn’t directly support element-wise multiplication of lists, you can achieve this functionality through list comprehensions or libraries like NumPy.
# Element-wise multiplication of lists
a = [1, 2, 3]
b = [4, 5, 6]
result = [a[i] * b[i] for i in range(len(a))]
print(result) # Output: [4, 10, 18]
4. Division (/) operator:
In Python, the division operator is represented by a forward slash (/
). It is used to divide one number (the dividend) by another (the divisor), resulting in a quotient. Unlike some other programming languages, Python’s division operator always performs floating-point division when dealing with integers, meaning it will return a float even if the division is evenly divisible. Here are five examples that illustrate the use of the division operator in various contexts.
4.1. Basic Division
The most straightforward use of the division operator is to divide two numbers.
# Dividing two integers
result = 8 / 2
print(result) # Output: 4.0
# Dividing a float by an integer
result = 9.0 / 3
print(result) # Output: 3.0
#Comment: Even though 8 / 2 is evenly divisible, the result is presented as a float (4.0) instead of an integer.
4.2. Division Resulting in a Floating-Point Number
When the division is not evenly divisible, the result is a floating-point number, providing a more precise answer.
# Dividing two integers
result = 7 / 2
print(result) # Output: 3.5
#This example demonstrates that Python's division operator provides the exact quotient without rounding or truncating the result.
4.3. Dividing by Zero
Attempting to divide by zero using the division operator results in a ZeroDivisionError
, as division by zero is undefined.
# Attempt to divide by zero
try:
result = 5 / 0
except ZeroDivisionError:
print("Cannot divide by zero!")
#This example uses a try-except block to catch the ZeroDivisionError and print a more user-friendly message.
4.4. Combining Division with Other Operators
The division operator can be combined with other arithmetic operators in expressions. Operator precedence determines the order in which operations are performed, but parentheses can be used to override this order.
# Combining division with addition
result = (2 + 3) / 5
print(result) # Output: 1.0
# Combining division with multiplication
result = 2 * 3 / 4
print(result) # Output: 1.5
#These examples demonstrate how to use parentheses to control the order of operations and how division interacts with other operators.
4.5. Division in Complex Expressions
The division operator can be part of more complex expressions, such as those involving variables and function calls.
# Using division in a complex expression
def average(a, b, c):
return (a + b + c) / 3
result = average(9, 6, 3)
print(result) # Output: 6.0
##This example defines a function that calculates the average of three numbers. It uses the division operator to divide the sum of the numbers by the count, demonstrating how division can be used in practical applications like calculating averages.
5. Modulus (%) Operator in Python:
The modulus operator, denoted by %
, is one of the arithmetic operators in Python that might not be as straightforward as addition or multiplication for beginners. However, it’s incredibly useful in various programming scenarios. The modulus operator returns the remainder of dividing the left-hand operand by the right-hand operand. In this post, we’ll explore 5 practical examples to understand how and when to use the modulus operator in Python.
5.1. Determining Even or Odd Numbers
One of the most common uses of the modulus operator is to determine whether a number is even or odd. If a number modulo 2 equals 0, it’s even; otherwise, it’s odd.
# Example 1: Checking if a number is even or odd
number = 7
if number % 2 == 0:
print(f"{number} is even.")
else:
print(f"{number} is odd.")
# Output: 7 is odd.
5.2. Cycling Through a List
When dealing with lists, sometimes you want to cycle through the list indexes in a loop. The modulus operator can help achieve this by wrapping around when the index exceeds the list length.
# Example 2: Cycling through a list
colors = ['red', 'green', 'blue']
for i in range(7): # Let's cycle through the list 7 times
print(colors[i % len(colors)])
# Output: red, green, blue, red, green, blue, red
5.3. Implementing a Simple Clock
In a scenario where you’re simulating a clock, the modulus operator can be used to wrap around the hours, minutes, or seconds when they exceed their maximum values.
# Example 3: Implementing a simple clock
hours = 23
minutes = 59
seconds = 58
for _ in range(5): # Simulate 5 seconds passing
seconds += 1
if seconds % 60 == 0:
seconds = 0
minutes += 1
if minutes % 60 == 0:
minutes = 0
hours = (hours + 1) % 24
print(f"{hours:02d}:{minutes:02d}:{seconds:02d}")
# Output: 23:59:59, 00:00:00, 00:00:01, 00:00:02, 00:00:03
5.4. Finding Factors of a Number
The modulus operator can be used to find factors of a given number by checking the remainder of division with each number in a certain range.
# Example 4: Finding factors of a number
number = 36
factors = []
for i in range(1, number + 1):
if number % i == 0:
factors.append(i)
print(f"Factors of {number}: {factors}")
# Output: Factors of 36: [1, 2, 3, 4, 6, 9, 12, 18, 36]
5.5. Creating a Custom Hash Function
While Python has built-in hash functions, understanding how to create a simple custom hash function using the modulus operator can be a great learning exercise. This can be particularly useful for implementing data structures like hash tables.
# Example 5: Creating a custom hash function
def simple_hash(key, table_size):
return key % table_size
keys = [10, 20, 30, 40, 50]
table_size = 8
hashes = [simple_hash(key, table_size) for key in keys]
print(f"Hashes: {hashes}")
# Output: Hashes: [2, 4, 6, 0, 2]
2. Comparison Operators:
Comparison operators in Python are used to compare two values. They evaluate to either True
or False
depending on the condition being tested. These operators are fundamental in making decisions and controlling the flow of programs through conditional statements like if
, elif
, and else
. Here are five key comparison operators, along with examples to illustrate their usage.
1. Equal to (==
)
The ==
operator checks whether the values on either side are equal. If they are, the expression evaluates to True
; otherwise, it evaluates to False
.
# Comparing integers
result = (5 == 5)
print(result) # Output: True
# Comparing strings
result = ("Python" == "python")
print(result) # Output: False
2. Not Equal to (!=
)
The !=
operator checks if the values on either side are not equal. If they are not equal, the expression evaluates to True
; if they are equal, it evaluates to False
.
# Comparing integers
result = (3 != 2)
print(result) # Output: True
# Comparing strings
result = ("Hello" != "hello")
print(result) # Output: True
3. Greater Than (>
)
The >
operator checks if the value on the left side is greater than the value on the right side. If it is, the expression evaluates to True
; otherwise, it evaluates to False
.
# Comparing numbers
result = (10 > 5)
print(result) # Output: True
# Comparing characters (based on ASCII values)
result = ('b' > 'a')
print(result) # Output: True
4. Less Than (<
)
The <
operator checks if the value on the left side is less than the value on the right side. If it is, the expression evaluates to True
; otherwise, it evaluates to False
.
# Comparing numbers
result = (2 < 5)
print(result) # Output: True
# Comparing characters
result = ('a' < 'b')
print(result) # Output: True
5. Greater Than or Equal to (>=
) and Less Than or Equal to (<=
)
The >=
operator checks if the value on the left side is greater than or equal to the value on the right side. Similarly, the <=
operator checks if the value on the left side is less than or equal to the value on the right side.
# Greater than or equal to
result = (5 >= 5)
print(result) # Output: True
result = (6 >= 2)
print(result) # Output: True
# Less than or equal to
result = (3 <= 5)
print(result) # Output: True
result = (4 <= 4)
print(result) # Output: True
3. Assignment Operators in Python
Assignment operators in Python are used to assign values to variables. While the basic assignment operator is the equals sign (=
), there are several augmented assignment operators that perform an operation on a variable and then assign the result back to that variable. These operators can make code more concise and readable. Here are five examples of assignment operators, illustrating their usage in Python.
1. Basic Assignment Operator (=
)
The equals sign (=
) is the basic assignment operator in Python. It assigns the value on the right to the variable on the left.
# Assigning an integer value to a variable
number = 10
print(number) # Output: 10
# Assigning a string value to a variable
message = "Hello, Python!"
print(message) # Output: Hello, Python!
2. Addition Assignment Operator (+=
)
The addition assignment operator (+=
) adds the value on the right to the variable on the left and then assigns the result back to the variable on the left.
# Adding and assigning in one step
counter = 5
counter += 3 # Equivalent to: counter = counter + 3
print(counter) # Output: 8
3. Subtraction Assignment Operator (-=
)
The subtraction assignment operator (-=
) subtracts the value on the right from the variable on the left and then assigns the result back to the variable on the left.
# Subtracting and assigning in one step
balance = 100
balance -= 30 # Equivalent to: balance = balance - 30
print(balance) # Output: 70
4. Multiplication Assignment Operator (*=
)
The multiplication assignment operator (*=
) multiplies the variable on the left by the value on the right and then assigns the result back to the variable on the left.
# Multiplying and assigning in one step
quantity = 4
quantity *= 2 # Equivalent to: quantity = quantity * 2
print(quantity) # Output: 8
5. Division Assignment Operator (/=
)
The division assignment operator (/=
) divides the variable on the left by the value on the right and then assigns the result back to the variable on the left. Note that this operator always performs floating-point division in Python 3.
# Dividing and assigning in one step
total = 100
total /= 4 # Equivalent to: total = total / 4
print(total) # Output: 25.0
4. Logical Operators
Logical operators in Python are used to combine conditional statements. They are fundamental in decision-making processes where multiple conditions must be evaluated to determine the outcome. Python supports three logical operators: and, or, and not. Here are examples illustrating the use of each logical operator.
1. The AND Operator
The and operator returns True if both the operands (conditions) are true, otherwise it returns False. It is used when you want to check if multiple conditions are true simultaneously.
# Example of the `and` operator
age = 25
membership = True
# Checking if age is greater than 18 and membership is True
if age > 18 and membership:
print("Eligible for discount")
else:
print("Not eligible for discount")
# Output: Eligible for discount
2. The or Operator
The or operator returns True if at least one of the operands (conditions) is true. It is used when you want to check if any one of multiple conditions is true.
# Example of the `or` operator
rainy = False
haveUmbrella = True
# Checking if it's raining or if you have an umbrella
if rainy or haveUmbrella:
print("You won't get wet")
else:
print("You might get wet")
# Output: You won't get wet
3. The not Operator
The not operator is used to invert the truth value. If a condition is true, applying the not operator makes it false, and vice versa.
# Example of the `not` operator
lightOn = True
# Checking if the light is not on
if not lightOn:
print("The room is dark")
else:
print("The room is lit")
# Output: The room is lit
4. Combining AND and OR
Logical operators can be combined to form complex conditions. When doing so, it’s essential to understand the precedence of operations or use parentheses to ensure the desired evaluation order.
# Combining `and` and `or`
age = 20
membership = False
coupon = True
# Checking if age is greater than 18 and either membership is True or coupon is True
if age > 18 and (membership or coupon):
print("Eligible for discount")
else:
print("Not eligible for discount")
# Output: Eligible for discount
5. Using not with AND and OR
The not operator can be combined with AND and OR to negate complex conditions.
# Using `not` with `and` and `or`
sunny = False
weekend = True
# Checking if it's not sunny and it's the weekend
if not sunny and weekend:
print("Good day for indoor activities")
else:
print("Let's go outside")
# Output: Good day for indoor activities
5. Identity Operators
Identity operators in Python are used to compare the memory locations of two objects. There are two identity operators: is
and is not
. The is
operator returns True
if two variables point to the same object, while the is not
operator returns True
if two variables do not point to the same object. Understanding identity operators is crucial when you want to check if two variables refer to the same object in memory, rather than just having the same value. Here are five examples illustrating the use of identity operators in Python.
1. Using the is
Operator
The is
operator checks if two variables refer to the same object in memory.
# Example of the `is` operator
list1 = [1, 2, 3]
list2 = list1 # list2 is referring to the same list as list1
result = (list1 is list2)
print(result) # Output: True
2. Using the “is not
” Operator
The is not
operator checks if two variables refer to different objects in memory.
# Example of the `is not` operator
string1 = "hello"
string2 = "hello"
result = (string1 is not string2)
print(result) # Output: False
3. Comparing Custom Objects
Identity operators can also be used to compare custom objects.
# Comparing custom objects
class Example:
pass
obj1 = Example()
obj2 = Example()
obj3 = obj1
print(obj1 is obj2) # Output: False
print(obj1 is obj3) # Output: True
4. is
with None
The is
operator is commonly used to check if a variable is None
.
# Checking if a variable is `None`
value = None
if value is None:
print("value is None")
else:
print("value is not None")
# Output: value is None
5. Using is not
with Collections
You can use the is not
operator to check if collections like lists, dictionaries, sets, etc., are not the same object in memory, even if they contain the same elements.
# Using `is not` with collections
list1 = [1, 2, 3]
list2 = [1, 2, 3]
print(list1 is list2) # Output: False
print(list1 == list2) # Output: True
Conclusion
Understanding and mastering the use of operators in Python is crucial for any programmer. They form the basis of all Python computations and logic. Through these 10 examples, we’ve seen how operators can be used to perform arithmetic operations, make comparisons, assign values, and evaluate logical conditions. Practice these examples and experiment with them to deepen your understanding of Python operators. Happy coding! You may like to read more here. The first lesson is here.
Further reading: