Your first python programs (10 examples)
Let’s start coding your first python programs (10 examples). Embarking on the journey of learning Python is an exciting venture. Python, known for its simplicity and versatility, has become one of the most popular programming languages in the world. Whether you aim to delve into web development, data analysis, artificial intelligence, or simply automate mundane tasks, Python is a great starting point. In this blog post, we’ll guide you through writing your very first Python program. By the end, you’ll have a solid understanding of some basic concepts and you’ll have written a simple, yet fully functional Python program. Let’s dive into your first python programs (10 examples).
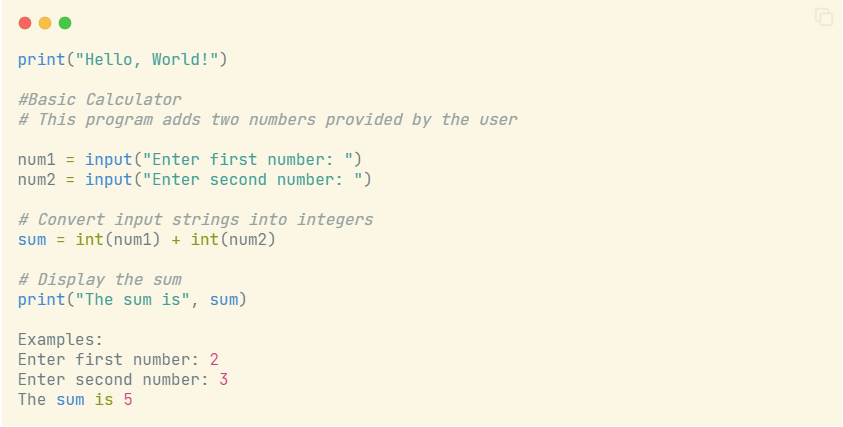
1. Writing your first python program:
The traditional first program in any programming language is “Hello, World!” It’s a simple program that outputs “Hello, World!” to the screen. This program is a great way to ensure your programming environment is set up correctly and gives you a taste of writing and executing a Python script. For Your first python programs, we are giving examples.
Open your favorite text editor or IDE (Integrated Development Environment) and type the following code:
print("Hello, World!")
#Basic Calculator
# This program adds two numbers provided by the user
num1 = input("Enter first number: ")
num2 = input("Enter second number: ")
# Convert input strings into integers
sum = int(num1) + int(num2)
# Display the sum
print("The sum is", sum)
Examples:
Enter first number: 2
Enter second number: 3
The sum is 5
Save your file with a .py
extension, for example, hello_world.py
. This extension tells your computer that this is a Python file.
To run your program, open your command line or terminal, navigate to the directory where your hello_world.py
file is saved, and type:
2. To print each character of a string on a new line
This is one the examples of your first python programs.
input_string = input("Enter a string: ")
for character in input_string:
print(character)
#Example
Enter a string: Safa
S
a
f
a
3. To print numbers from 1 to 5
This one of the examples of Your first python programs:
for number in range(1, 6):
print(number)
1
2
3
4
5
4. Print Multiplication Table
Creating a simple multiplication table should an example of Your first python programs. Creating a Python program to print the multiplication table for a given number is a straightforward task that involves using a for
 loop to iterate through the numbers 1 to 10 (or any range you choose) and multiplying them by the given number. Here’s a simple example:
# Ask the user to input the number for which they want the multiplication table
number = int(input("Enter the number for which you want the multiplication table: "))
# Define the range of the multiplication table (1 to 10 in this case)
for i in range(1, 11):
# Calculate the product
product = number * i
# Print the multiplication fact
print(f"{number} x {i} = {product}")
Example:
Enter the number for which you want the multiplication table: 5
5 x 1 = 5
5 x 2 = 10
5 x 3 = 15
5 x 4 = 20
5 x 5 = 25
5 x 6 = 30
5 x 7 = 35
5 x 8 = 40
5 x 9 = 45
5 x 10 = 50
Note:
This program first prompts the user to enter a number. It then uses a for
 loop to iterate through the numbers 1 to 10. In each iteration, it calculates the product of the given number and the iterator (i
), and prints the result in a formatted string that represents a line of the multiplication table.
5. Customize the range of Multiplication Table
If you want to extend the range of the multiplication table beyond 1 to 10, you can modify the range()
 function in the for
 loop. For example, to print the multiplication table up to 20, change the range(1, 11)
 to range(1, 21)
:
number = int(input("Enter the number for which you want the multiplication table: "))
for i in range(1, 21): # Now goes up to 20
print(f"{number} x {i} = {number * i}")
#Example
Enter the number for which you want the multiplication table: 2
2 x 1 = 2
2 x 2 = 4
2 x 3 = 6
2 x 4 = 8
2 x 5 = 10
2 x 6 = 12
2 x 7 = 14
2 x 8 = 16
2 x 9 = 18
2 x 10 = 20
2 x 11 = 22
2 x 12 = 24
2 x 13 = 26
2 x 14 = 28
2 x 15 = 30
2 x 16 = 32
2 x 17 = 34
2 x 18 = 36
2 x 19 = 38
2 x 20 = 40
6. Print Multiplication Table in a File
You might also want to save the multiplication table to a file instead of just printing it to the console. Here’s how you can do it:
number = int(input("Enter the number for which you want the multiplication table: "))
# Open a file in write mode
with open(f"Multiplication_Table_of_{number}.txt", "w") as file:
for i in range(1, 11):
file.write(f"{number} x {i} = {number * i}\n")
print(f"Multiplication table of {number} has been saved to 'Multiplication_Table_of_{number}.txt'")
#To create a link of the "Multiplication Table of Your Number, you can write the following code:
from IPython.display import FileLink
FileLink(r'Multiplication_Table_of_5.txt')
#After writin this code, you will see a link to download the text file. See the image below:
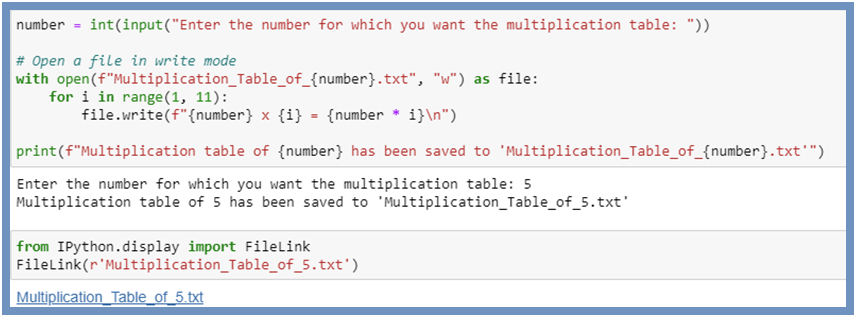
7. Check Prime Numbers
This program checks if a number entered by the user is a prime number or not.
num = int(input("Enter a number: "))
# Prime numbers are greater than 1
if num > 1:
for i in range(2, num):
if (num % i) == 0:
print(num, "is not a prime number")
break
else:
print(num, "is a prime number")
else:
print(num, "is not a prime number")
#Example 1
Enter a number: 5
5 is a prime number
#Example 2
Enter a number: 8
8 is not a prime number
8. calculate Factorials
This example calculates the factorial of a given number using a loop.
num = int(input("Enter a number: "))
factorial = 1
if num < 0:
print("Sorry, factorial does not exist for negative numbers")
elif num == 0:
print("The factorial of 0 is 1")
else:
for i in range(1, num + 1):
factorial = factorial * i
print("The factorial of", num, "is", factorial)
#Example
Enter a number: 5
The factorial of 5 is 120
9. Find the Sum of Natural Numbers
This program calculates the sum of the first n
 natural numbers, where n
 is provided by the user. Creating a simple program to count the sum of the first n natural numbers should be one of your first python programs.
n = int(input("Enter a number: "))
if n < 0:
print("Enter a positive number")
else:
sum = 0
# use while loop to iterate until zero
while(n > 0):
sum += n
n -= 1
print("The sum is", sum)
#Example
Enter a number: 5
The sum is 15
10. Calculate Square Roots
This example calculates the square root of a number entered by the user.
num = float(input("Enter a number: "))
sqrt = num ** 0.5
print("The square root of", num, "is", sqrt)
#Example
Enter a number: 144
The square root of 144.0 is 12.0
Conclusion
Writing your first Python program is a significant milestone. Today, you learned how to set up your Python environment, write a simple program, and expand it to interact with the user. These are foundational skills that you will build upon as you continue to learn Python and explore its vast ecosystem.
One Response