Mastering Variables and Data Types in Python: 10 Examples
In this tutorial, we discuss about “Mastering Variables and Data Types in Python: 10 Examples“. Python, known for its simplicity and readability, is a favorite among both beginners and seasoned developers. Central to its ease of use are variables and data types, which allow for efficient data storage and manipulation. This guide will delve into the core concepts of variables and data types in Python, accompanied by 10 practical examples to solidify your understanding. In this tutorial, we will discuss on mastering variable types and data types in python: 10 examples. If you want to have an idea about the first python programs, you may like to read “My First Python Programs”.
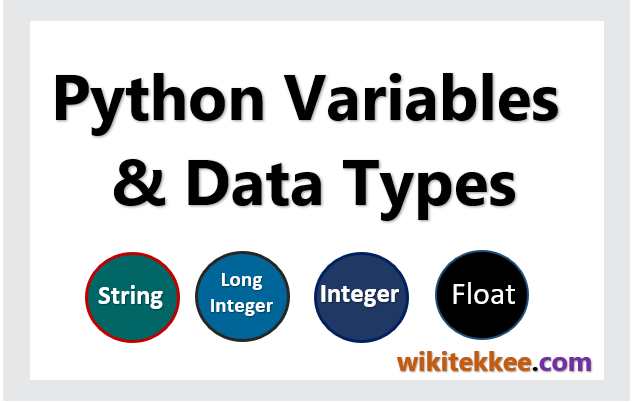
1. Understanding Variables in Python
In our discussion on “Mastering Variables and Data Types in Python: 10 Examples“, we first discuss about the basics of variables. Variables in Python are like labels that you can attach to values. They don’t actually contain the data but rather reference a location in memory where the data is stored. This makes variables extremely versatile, as you can change the value they reference at any time.
Declaration and Assignment
In Python, declaring a variable and assigning it a value is as simple as:
variable_name = value
Python uses dynamic typing, which means you don’t need to explicitly state the type of the variable. The type is inferred from the value it is assigned.
2. Exploring Python Data Types
Mastering Variables and Data Types in Python: 10 Examples. Python offers a variety of data types, allowing you to work with numbers, text, boolean values, and complex collections of data. Here are the primary data types:
- Integer (
int
): Whole numbers, positive or negative. - Float (
float
): Numbers with a decimal point. - String (
str
): A sequence of characters. - Boolean (
bool
): RepresentsTrue
orFalse
. - List (
list
): An ordered collection of items. - Tuple (
tuple
): Similar to a list, but immutable. - Dictionary (
dict
): A collection of key-value pairs. - Set (
set
): An unordered collection of unique elements.
3. Integer Variables in Python
An integer variable in Python is declared simply by assigning a whole number to a variable name. Python uses dynamic typing, which means you don’t have to explicitly declare the type of the variable; it’s inferred from the value.
Example 1: Declaring an Integer Variable
age = 25 print(age) # Output: 25
In this example, age
 is a variable of type integer with a value of 25.
Characteristics of Python Integers
- Whole Numbers: Integers can represent whole numbers without a fractional component, ranging from negative to positive infinity, limited only by the available memory.
- Immutability: Integers in Python are immutable, meaning that once an integer object is created, its value cannot be changed. Any operation that modifies an integer value results in the creation of a new integer object.
- Arbitrary Precision: Python integers have unlimited precision, which means they can grow to hold any number of digits as required by your calculations. This is a significant advantage over languages that limit integer sizes, potentially leading to overflow errors.
- Base Representations: Python allows integers to be represented in decimal (base 10), binary (base 2), octal (base 8), and hexadecimal (base 16) formats.
Example 2: Immutability of Integers
x = 10
print(id(x)) # Unique identifier for the object referred to by x
# Attempt to modify x
x += 1
print(id(x)) # The identifier has changed, indicating a new object
Example 3: Arbitrary Precision
huge_number = 1234567890123456789012345678901234567890
print(huge_number) # Output: 1234567890123456789012345678901234567890
Example 4: Base Representations
# Binary representation
binary_number = 0b1010 # Equivalent to decimal 10
print(binary_number) # Output: 10
# Octal representation
octal_number = 0o12 # Equivalent to decimal 10
print(octal_number) # Output: 10
# Hexadecimal representation
hex_number = 0xA # Equivalent to decimal 10
print(hex_number) # Output: 10
Operations with Integer Variables:
Python supports a wide range of operations with integers, including arithmetic, comparison, and bitwise operations.
Example 5: Arithmetic Operations
a = 10
b = 3
print(a + b) # Addition: 13
print(a - b) # Subtraction: 7
print(a * b) # Multiplication: 30
print(a / b) # Division (results in a float): 3.333...
print(a // b) # Integer division: 3
print(a % b) # Modulus (remainder): 1
print(a ** b) # Exponentiation: 1000
Example 6: Comparison Operations
x = 5
y = 10
print(x == y) # Equality: False
print(x != y) # Inequality: True
print(x < y) # Less than: True
print(x > y) # Greater than: False
Example 7: Bitwise Operations
x = 2 # 0b10 in binary
y = 3 # 0b11 in binary
print(x & y) # Bitwise AND: 2 (0b10)
print(x | y) # Bitwise OR: 3 (0b11)
print(x ^ y) # Bitwise XOR: 1 (0b01)
print(~x) # Bitwise NOT: -3 (inverts all bits)
print(x << 1) # Left shift: 4 (0b100)
print(x >> 1) # Right shift: 1 (0b1)
Integers are a versatile and essential data type in Python, supporting a wide range of operations that enable complex calculations and logic to be performed efficiently. By understanding the characteristics and capabilities of integer variables, you can harness the full power of Python for numerical computing and beyond.
4. Understanding float Variables in Python
In Python, floating-point numbers, or simply “floats,” are used to represent real numbers that include a fractional part. These numbers are crucial for performing arithmetic that requires precision beyond whole numbers. This guide will delve into the intricacies of float variables in Python, backed by five practical examples to enhance your understanding.
Understanding Float Variables
Float variables in Python are created by assigning a number with a decimal point to a variable. Python automatically recognizes numbers containing a decimal point as floats. It’s important to note that floats in Python are implemented using double precision (64-bit) according to the IEEE 754 standard, which affects their precision and storage.
Example 1: Declaring a Float Variable
temperature = 36.5 print(temperature) # Output: 36.5
In this example, temperature
 is a float variable representing a numeric value with a fractional part.
Characteristics of Float Variables
- Precision: Floats have a precision limit. While they can represent very large or very small numbers, the number of significant digits is limited to about 15 decimal places. This can lead to rounding errors in complex calculations.
- Scientific Notation: Python allows float values to be expressed in scientific notation for very large or very small numbers, improving readability and precision.
- Arithmetic Operations: Floats support all standard arithmetic operations, but mixing floats with integers in operations automatically converts the result to a float.
- Immutability: Similar to integers, float variables in Python are immutable. Any operation that alters the value of a float results in the creation of a new float object.
Example 2: Float from Division
average = 7 / 2
print(average)
In this example, the result of the division 7 / 2
 is a float (3.5
), which is assigned to the variable average
. Python automatically converts the division result to a float.
Example 3: Scientific Notation
speed_of_light = 3.0e8
print(speed_of_light)
Here, speed_of_light
 is assigned a float value using scientific notation. 3.0e8
 stands for (3.0 \times 10^8), which is (300,000,000.0).
Example 4: Converting Integer to Float
num_int = 10
num_float = float(num_int)
print(num_float)
This example demonstrates converting an integer to a float using the float()
 function. num_int
 is an integer that gets converted to a float num_float
, which is 10.0
 when printed.
Example 5: Float in Complex Calculation
result = 0.1 + 0.2 - 0.3
print(result)
This might seem straightforward, but due to how floats are represented in the computer’s memory, the result might not be exactly 0.0
as expected, but very close to it, demonstrating the precision aspect of float operations.
Float variables are essential in Python for handling numerical data that requires decimal points. They are widely used in various applications such as scientific calculations, financial computations, and anywhere else that precise numerical representation is necessary. Mastering Variables and Data Types in Python: 10 Examples
5. Understanding String Variables in Python
String variables in Python are used to store sequences of characters. These characters can include letters, numbers, symbols, and spaces. Python treats single quotes ('
) and double quotes ("
) interchangeably when defining strings, allowing for flexibility in incorporating quotes and other characters within the string itself.
Here a
String variables in Python are used to store sequences of characters. These characters can include letters, numbers, symbols, and spaces. Python treats single quotes ('
) and double quotes ("
) interchangeably when defining strings, allowing for flexibility in incorporating quotes and other characters within the string itself.
Here are 5 examples of operations that can be performed with string variables in Python:
Example 1: Concatenation
Concatenation is the process of joining two or more strings together.
first_name = "John" last_name = "Doe" full_name = first_name + " " + last_name print(full_name)
Output: John Doe
Example 2: Repetition
Strings can be repeated a specified number of times using the *
operator.
repeat_str = "Ha" laugh = repeat_str * 3 print(laugh)
Output: HaHaHa
Example 3: Slicing
Slicing is used to extract a substring from a string by specifying the start and end indices.
text = "Hello, World!" sub_text = text[7:12] print(sub_text)
Output: World
Example 4: Changing Case
Python provides methods to change the case of the text in a string, such as converting to uppercase or lowercase.
original_text = "Python Programming" upper_text = original_text.upper() lower_text = original_text.lower() print("Uppercase:", upper_text) print("Lowercase:", lower_text)
Output:
Uppercase: PYTHON PROGRAMMING
Lowercase: python programming
Example 5: Finding Substrings
The find()
method returns the lowest index of the substring if it is found in the string. If it’s not found, it returns -1
.
quote = "The quick brown fox jumps over the lazy dog" position = quote.find("fox") print("Position of 'fox':", position)
Output: Position of 'fox': 16
These examples illustrate just a few of the many operations that can be performed with string variables in Python. Strings are incredibly versatile and powerful, playing a critical role in almost every Python application, from simple scripts to complex systems. They are used for handling text, formatting data, generating output, and much more.
6. Understanding Boolean (bool
) Variables in Python
In this discussion on “Mastering Variables and Data Types in Python: 10 Examples“, we also discuss on Boolean. Boolean variables in Python are used to represent two values:Â True
 or False
. These are the only two values of the built-in type bool
. Boolean variables are extensively used in conditional statements and loops as they help in decision-making processes by evaluating conditions as either True
 or False
. Here are 5 examples illustrating the use and manipulation of Boolean variables in Python:
Example 1: Basic Boolean Assignment
This example demonstrates the basic assignment of Boolean values to variables.
is_active = True is_closed = False print("Is active:", is_active)
print("Is closed:", is_closed)
Example 2: Comparison Operations
Boolean values are commonly the result of comparison operations.
a = 10
b = 20
result = (a > b) # This will be False because 10 is not greater than 20 print("Is a greater than b?", result)
Output: Is a greater than b? False
Example 3: Logical Operations
Logical operators (and
, or
, not
) are used to combine conditional statements and return Boolean values.
x = True
y = False
print("x and y:", x and y) # False, because both are not True print("x or y:", x or y) # True, because at least one is True print("not x:", not x)
# False, because x is True
Example 4: Boolean in Conditional Statements
Booleans are pivotal in the flow control of programs, particularly in if-else statements.
age = 18 if age >= 18: adult = True else: adult = False print("Is adult:", adult)
Output: Is adult: True
This example uses a Boolean variable to store the result of a condition that checks if someone is an adult based on their age.
Example 5: Boolean Values from Functions
Functions can return Boolean values, which is useful for checking conditions or states.
def is_even(number):
return number % 2 == 0 print("Is 10 even?", is_even(10))
# True print("Is 5 even?", is_even(5)) # False
These examples showcase the versatility and importance of Boolean variables in Python programming. They are fundamental in controlling the flow of programs, performing comparisons, and handling conditions.
7. Understanding Python Lists
Python lists are versatile, ordered collections of items (elements). Each item in a list can be of any data type, and a single list can even contain items of different data types. Lists are mutable, meaning their contents can be changed after creation. They are defined by enclosing items within square brackets []
.
Here are 5 examples illustrating various operations with Python lists:
Example 1: Creating and Accessing a List
# Creating a list
fruits = ["apple", "banana", "cherry"]
# Accessing elements
print(fruits[0]) # Output: apple
print(fruits[2]) # Output: cherry
# Negative indexing
print(fruits[-1]) # Output: cherry (last item)
Example 2: Adding and Removing Elements
# Adding elements
fruits.append("orange") # Adds "orange" to the end
fruits.insert(1, "mango") # Inserts "mango" at position 1
# Removing elements
fruits.remove("banana") # Removes "banana"
popped_fruit = fruits.pop() # Removes and returns the last item ("orange")
print(fruits) # Output: ['apple', 'mango', 'cherry']
Example 3: List Slicing
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
# Slicing
first_three = numbers[:3] # Gets the first three elements
last_three = numbers[-3:] # Gets the last three elements
middle = numbers[3:7] # Gets elements from index 3 to 6
print("First three:", first_three) # Output: [0, 1, 2]
print("Last three:", last_three) # Output: [7, 8, 9]
print("Middle:", middle) # Output: [3, 4, 5, 6]
Example 4: List Comprehensions
List comprehensions provide a concise way to create lists based on existing lists.
# Creating a list of squares using list comprehension
squares = [x**2 for x in range(10)]
print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Example 5: Sorting and Reversing
# Sorting a list
ages = [42, 24, 35, 18, 30]
ages.sort() # Sorts in ascending order
print("Sorted ages:", ages) # Output: [18, 24, 30, 35, 42]
# Reversing a list
ages.reverse() # Reverses the list
print("Reversed ages:", ages) # Output: [42, 35, 30, 24, 18]
Python lists are incredibly flexible and powerful, serving as a foundation for many data structures and algorithms. They are used for storing collections of data, iterating over sequences, and much more, making them an essential part of Python programming.
8. Understanding Tuples in Python
A tuple in Python is an ordered collection of items, similar to a list. The key differences between tuples and lists are that tuples are immutable (they cannot be changed after creation) and are defined by enclosing the elements in parentheses ()
instead of square brackets []
. Tuples can contain elements of different data types, making them a versatile option for grouping data.
Here are 5 examples illustrating various operations and uses of tuples in Python:
Example 1: Creating and Accessing a Tuple
# Creating a tuple
coordinates = (10.0, 20.0)
# Accessing tuple elements
print(coordinates[0]) # Output: 10.0
print(coordinates[1]) # Output: 20.0
# Nested tuple
nested_tuple = ("apple", (1, 2, 3), ["a", "b", "c"])
print(nested_tuple[1]) # Output: (1, 2, 3)
Example 2: Tuple Unpacking
Tuple unpacking allows you to assign each item in a tuple to a variable in a single statement.
point = (3, 5)
x, y = point
print("x:", x) # Output: x: 3
print("y:", y) # Output: y: 5
Example 3: Tuples in Functions
Tuples can be used to return multiple values from a function.
def min_max(numbers):
return min(numbers), max(numbers) # Returns a tuple
result = min_max([1, 2, 3, 4, 5])
print("Min and max:", result) # Output: Min and max: (1, 5)
Example 4: Tuple Concatenation and Repetition
Tuples support concatenation and repetition operations, similar to strings and lists.
tuple1 = (1, 2, 3)
tuple2 = (4, 5, 6)
# Concatenation
concatenated = tuple1 + tuple2
print("Concatenated tuple:", concatenated) # Output: Concatenated tuple: (1, 2, 3, 4, 5, 6)
# Repetition
repeated = tuple1 * 2
print("Repeated tuple:", repeated) # Output: Repeated tuple: (1, 2, 3, 1, 2, 3)
Example 5: Tuple Membership Test
You can check if an item exists in a tuple using the in
 operator.
fruits = ("apple", "banana", "cherry")
print("apple" in fruits) # Output: True
print("orange" in fruits) # Output: False
These examples showcase the versatility and utility of tuples in Python. Despite their immutability, tuples are widely used in situations where a sequence of values needs to be protected from modification, or when returning multiple values from functions. Their immutability also makes them hashable, allowing them to be used as keys in dictionaries, among other uses.
9. Understanding Python Dictionary
A Python dictionary (dict
) is a collection of key-value pairs, where each key is unique and is used to access its corresponding value. Dictionaries are mutable, meaning their contents can be changed after they are created. They are defined by enclosing key-value pairs in curly braces {}
, with each key separated from its value by a colon :
. Dictionaries are optimized for retrieving values when the key is known. Here are 5 examples illustrating various operations and uses of dictionaries in Python:
Example 1: Creating and Accessing a Dictionary
# Creating a dictionary
person = {"name": "John", "age": 30, "city": "New York"}
# Accessing dictionary values
print(person["name"]) # Output: John
print(person.get("age")) # Output: 30
# Accessing a non-existent key (safe method)
print(person.get("profession", "Not specified")) # Output: Not specified
Example 2: Adding and Modifying Elements
# Adding a new key-value pair
person["profession"] = "Software Engineer"
# Modifying an existing key's value
person["city"] = "San Francisco"
print(person)
# Output: {'name': 'John', 'age': 30, 'city': 'San Francisco', 'profession': 'Software Engineer'}
Example 3: Removing Elements
# Removing a key-value pair using pop
profession = person.pop("profession")
print("Removed profession:", profession)
# Removing a key-value pair using del
del person["city"]
print(person) # Output: {'name': 'John', 'age': 30}
Example 4: Iterating Through a Dictionary
# Iterating through keys
for key in person.keys():
print(key)
# Iterating through values
for value in person.values():
print(value)
# Iterating through key-value pairs
for key, value in person.items():
print(key, ":", value)
Example 5: Dictionary Comprehensions
# Creating a dictionary of squares using dictionary comprehension
squares = {x: x**2 for x in range(6)}
print(squares)
# Output: {0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
These examples demonstrate the flexibility and utility of dictionaries in Python. They are widely used for storing and manipulating data in a structured way, enabling fast lookups by keys, and supporting various operations such as adding, modifying, and removing key-value pairs. Dictionaries are essential in Python for working with JSON data, managing configurations, and much more.
10. Understanding Python Set
A Python set is a collection of unique elements, meaning it does not allow duplicates. Sets are mutable, unordered, and are defined by enclosing elements within curly braces {}
. An empty set can be created using the set()
 constructor, as {}
 is used for dictionaries. Sets are particularly useful for performing mathematical set operations like union, intersection, difference, and symmetric difference. Here are 5 examples illustrating various operations and uses of sets in Python:
Example 1: Creating and Adding Elements to a Set
# Creating a set
fruits = {"apple", "banana", "cherry"}
# Adding elements
fruits.add("orange") # Adding a single element
print(fruits) # Output: {'banana', 'cherry', 'orange', 'apple'}
# Adding multiple elements (update)
fruits.update(["mango", "grape"])
print(fruits) # Output may vary due to unordered nature of sets
Example 2: Removing Elements from a Set
# Removing an element safely with discard (no error if element is not found)
fruits.discard("banana")
print(fruits)
# Removing an element with remove (raises KeyError if element is not found)
fruits.remove("apple")
print(fruits)
# Removing and returning an arbitrary element with pop()
popped_fruit = fruits.pop()
print("Popped fruit:", popped_fruit)
print(fruits)
Example 3: Set Operations
Sets support mathematical set operations like union, intersection, and difference.
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
# Union
print("Union:", set1 | set2) # Output: {1, 2, 3, 4, 5, 6, 7, 8}
# Intersection
print("Intersection:", set1 & set2) # Output: {4, 5}
# Difference
print("Difference:", set1 - set2) # Output: {1, 2, 3}
Example 4: Checking Membership
Sets can be used to efficiently check if an element exists within them due to their hash table implementations.
print("apple" in fruits) # Output: False (since "apple" was removed earlier)
print("mango" in fruits) # Output: True
Example 5: Set Comprehensions
# Creating a set of squares using set comprehension
squares = {x**2 for x in range(6)}
print(squares) # Output: {0, 1, 4, 9, 16, 25}
These examples illustrate the versatility and utility of sets in Python. Sets are particularly useful for handling collections of unique items, performing set operations, and for situations where the order of elements is not important. They provide a fast way to remove duplicates from a sequence and to check for membership due to their underlying hash-based implementation.
Conclusion
Understanding variables and data types is crucial for efficiently storing and manipulating data in Python programs. Python’s dynamic typing and rich set of built-in data types make it a flexible and powerful language for a wide range of applications.
# Creating variables
name = "John Doe" # A string variable
age = 30 # An integer variable
height = 5.9 # A floating-point variable
is_adult = True # A boolean variable
# Printing variables
print(name) # Output: John Doe
print(age) # Output: 30